Resources
- //PROCESS: Using function parameters and returns
- //PROCESS: Functions: Definition, trigger, or reference?
- //STYLE: Use function pipelines
Saving your work
Use Save as...
to save the
empty template as
"2.12S-ConvertYourCodeToFunctionPipelines-LastName.html
"
in your Computer Programming 12 directory.
The assignment
Here is what the initial program does:
You are my biggest star!
Press the U and D keys to make the star bigger and littler.
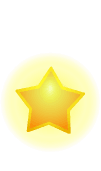
Now convert the sample program from a sequential program into one that uses function pipelines.
- Create an //INPUT function called readKeypress( keyEvent ) that returns the character that was pressed.
-
Give the function a descriptive comment and add
console.log( 'in readKeypress...' );
- Create an //OUTPUT function called makeStarSmaller()
- Create an //OUTPUT function called makeStarBigger()
-
Give each //OUTPUT function a descriptive comment and add
console.log()
s - Create a //PROCESS function called getBiggerOrSmaller( characterPressed )
-
Give the function a descriptive comment and add
console.log( 'in getBiggerOrSmaller...' );
-
Add the
if
statements that call eithermakeStarBigger()
ormakeStarBigger()
; - Now alter the code as you wish to make it interesting, but respect the seperation of input, processing, and output!
The beginning code
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Convert your code to function pipelines</title>
<meta
name="description"
content="Assignment: Convert your code to function pipelines">
<meta name="author" content="--yourName--">
<!--
Change log:
Created: 12. October 2015 - Drapak
Modified: 25. March 2017 - improved programming style - Drapak
30. April 2017 - fixed long lines - Drapak
21. October 2018 - updated style and clarity - Drapak
Finished:
-->
<!-- load jQuery for the animations -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js">
</script>
<style>
img {
width: 80px;
}
</style>
</head>
<body>
<h1>You are my biggest star!</h1>
<p>Press the U and D keys to make the star bigger and littler.</p>
<img
id="imageId"
alt="picture of a star"
src="https://www.kasandbox.org/programming-images/cute/Star.png">
<!--
Convert this sample program from a sequential program
into one that uses function pipelines.
1. Create an //INPUT function called readKeypress( keyEvent )
that returns the character that was pressed.
2. Give the function a descriptive comment and
add console.log( 'in readKeypress...' );
3. Create an //OUTPUT function called makeStarSmaller()
4. Create an //OUTPUT function called makeStarBigger()
5. Give each //OUTPUT function a descriptive comment and add console.log()s
6. Create a //PROCESS function called getBiggerOrSmaller( characterPressed )
7. Give the function a descriptive comment and
add console.log( 'in getBiggerOrSmaller...' );
8. Add the if statements that call either makeStarBigger() or makeStarBigger();
9. Now alter the code as you wish to make it interesting,
but respect the seperation of input, processing, and output!
------------STYLE GUIDE FOR LONG LINES: DO NOT PASS COLUMN 95 -------------------------------|
-->
<script>
//MAIN
var mainProcedure = function ( keyEvent ) {
console.log( 'in mainProcedure...' );
//INPUT: read which key was pressed
var unicodeNumber = keyEvent.which || keyEvent.keyCode;
//IF: the U key has been pressed
if ( unicodeNumber == 85 ) {
//OUTPUT: make the star bigger
$( '#imageId' ).animate( {width: "+=40px"} );
//IF: the D key has been pressed
} else if ( unicodeNumber == 68 ) {
//OUTPUT: make the star smaller
$( '#imageId' ).animate( { width: "-=40px" } );
}
}
//INPUT: run mainProcedure when someone presses a key
window.onkeydown = mainProcedure;
</script>
</body>
</html>